What is Instruction Set Architecture (ISA)?
Published on 2022-05-02
Category: Misc
“Instruction Set Architecture is the structure of a computer that a machine language programmer (or a compiler) must understand to write a correct (timing-independent) program for that machine.” — IBM introducing 360 (1964)
ISA can be seen as a bridge between hardware and software. It gathers the operations that a processor can execute and transfers them to the microarchitecture for further implementation.
Instruction Syntaxes
Instructions are what ISA uses to execute tasks. An instruction usually consists of an operator
(op
), source operands (src
), and a destination operand (dest
).
Common Syntax Examples:
- Intel x86:
op dest, src1, src2
- AT&T x86:
op src1, src2, dest
- MIPS:
op dest, src1, src2 sw src, dest
- ARM:
op register, src/dest
Arithmetic Logic Unit (ALU)
The ALU is a digital electronic circuit designed to perform arithmetic operations on integer source operands. These operands can originate from the Stack, Accumulator, Registers, or memory. After receiving operations from any of these sources, it produces a result.
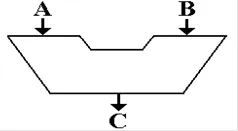
ISA Operands
As mentioned before, the ALU performs arithmetic operations on source operands provided by the Stack, Accumulator, Registers, or memory. Let's dive deeper into each one!
Stack
The stack acts as a source or a destination, and it uses a push/pop structure with one explicit operand. Let's use an example to illustrate this:
C = A + B
Think of the stack like a stack of pancakes. We push operand A onto the stack, then operand B. We then perform the addition operation, and the result is stored in C.
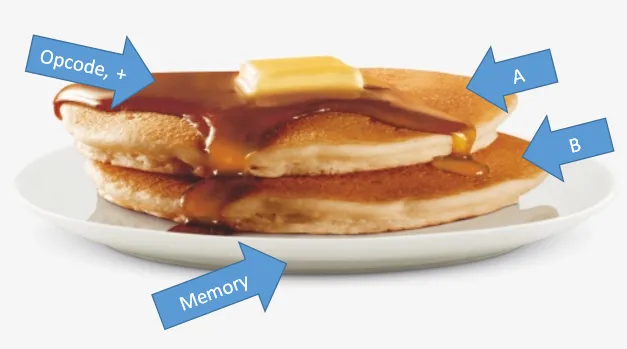
Accumulator
The accumulator is a single implicit register that acts as a source or destination. It can become a performance bottleneck due to its implicit implementation.
Using the example C = A + B again, A is loaded into the accumulator first, then B is added to it. The sum is then stored in C.
Register-Register/Load-Store
In this model, registers hold operands, but the actual values are stored in memory. Registers must load these values before sending them to the ALU. Most RISC architectures use this format.
Register-Memory
Operands are read from memory and registers. Most x86 architectures use this format, and operands can be anywhere in memory or registers. This format is common in CISC architectures.
Addressing Modes
Addressing modes specify how operands are loaded from memory or registers. Below are some common addressing modes with x86 format examples:
Register
Operands are located in registers.
add R1, R2, R3
Immediate
Uses a constant value as an operand.
add R1, R2, 6
Register Indirect/Deferred
The memory address is stored in a register, similar to using a pointer in programming.
add R1, R2, [R3]
Displacement
Combines a base address and an offset.
add R1, R2, [R3 + 200]
Indexed
Accesses array elements using an index stored in a register.
add R1, R2, [R3 + R4]
Two-Dimensional Array Example
Two-dimensional arrays can be tricky in assembly. Let's consider an array x[5][7]
with 10
elements per row and 8 bytes per element.
The offset calculation is:
Offset = Row * Number of Columns * Size of Element
Offset = 5 * 10 * 8 = 400 bytes
The instruction would look like:
add R1, R2, [R3 + R4 * 8 + 400]
Fixed-Length vs Variable-Length Instructions
Instructions can be encoded in fixed-length or variable-length formats.
Fixed-Length Instructions
Advantages:
- Easy to decode
- Uniform instruction size
Disadvantages:
- Consumes more memory
Variable-Length Instructions
Advantages:
- Efficient memory usage
Disadvantages:
- Complex decoding
CISC vs RISC Architecture
Complex Instruction Set Computer (CISC) aims to reduce the number of instructions per program while increasing the cycles per instruction. Reduced Instruction Set Computer (RISC) aims to reduce the cycles per instruction at the cost of increasing the number of instructions per program.
Most modern processors utilize RISC architecture. Examples include MIPS, RISC-V, and ARM. CISC architectures include x86 and VAX.
CISC Advantages
- Better programmability
- Smaller code sizes
- Backward compatibility
RISC Advantages
- Easier to implement
- Fewer transistors required
- Better energy efficiency
- Backward compatibility
SIMD
Single Instruction Multiple Data (SIMD) performs the same mathematical operation on multiple data points simultaneously using one instruction. It's used in MMX, 3DNow!, AVX, and SSE.
Five Stages of ISA Implementations
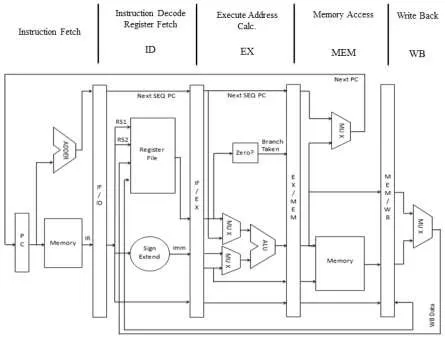
- Instruction Fetch (IF): The instruction is read from the program counter. Components: Adder, Memory Unit.
- Instruction Decode/Register Fetch (ID): Decodes the instruction and reads source operands. Components: Register File, Sign Extender.
- Execute/Address Calculation (EX): Performs arithmetic or logical operations. Components: ALU, Zero Flag.
- Memory Access (MEM): Accesses memory and completes branch instructions. Components: Memory Unit.
- Write-Back (WB): Writes results back into registers. Components: Register File.
Conclusion
ISA is the foundation of computer architecture, acting as the vital link between hardware and software. Understanding its components, addressing modes, and implementations is key to mastering the world of computing.